Note
Click here to download the full example code
Options for converting to mne.Raw
Set up
import os
import mne
from mkpy import mkh5
from mkpy.io import mkh5mne
mne.viz.set_browser_backend("matplotlib") # for docs generation
# FYI
conda_env = os.environ["CONDA_DEFAULT_ENV"]
print("conda env", conda_env)
for pkg in [mkh5, mne]:
print(pkg.__name__, pkg.__version__)
Out:
conda env env_3.8
mkpy.mkh5 0.2.7
mne 1.0.3
mkh5 data file format in a nutshell
This sample mkh5 data is for a single subject in an auditory oddball paradigm with previously set epoch tables.
H5_F = "../mkh5_data/sub000p3.h5"
h5_data = mkh5.mkh5(H5_F)
Stimulus and response events of interest have been tagged with an mkh5 codemap and stored
as named epochs tables mkh5.set_epochs(...)
. The epochs tables are whatever was deemed
useful.
h5_data.get_epochs_table_names()
# The epochs tables have index information about where to find the
# events in the mkh5 file, the experimental variables from
# the codemap, and the epoch discrete time interval specs, relative
# to the current mkh5 datablock.
epochs_table = h5_data.get_epochs_table("ms1500")
epochs_table[
[
"epoch_id",
"data_group",
"dblock_path", # HDF5 data lookup info
"log_evcodes",
"log_ccodes",
"log_flags", # event code info
"tone",
"stim",
"accuracy",
"acc_type", # codemap tags
"diti_t_0",
"diti_hop",
"diti_len", # epoch t_0, offset, duration
]
]
The mkh5 EEG and event channel data are stored in datablocks (HDF5 Dataset) at the end of an HDF5 “slash” path:
h5_data.dblock_paths
Out:
['sub000/dblock_0', 'sub000/dblock_1', 'sub000/dblock_2', 'sub000/dblock_3', 'sub000/dblock_4']
Electrode locations
Converting mkh5 to mne.Raw requires an mkh5 format apparatus map with the coordinate space, fiducal landmarks and electrode locations. If the appartus map was included with the YAML .yhdr when the mkh5 file was created it will be used automatically.
mne_raw = mkh5mne.from_mkh5(H5_F)
Out:
../mkh5_data/sub000p3.h5
looking up data block paths, larger files take longer ...
ok
checking info, montage sub000/dblock_1
checking info, montage sub000/dblock_2
checking info, montage sub000/dblock_3
checking info, montage sub000/dblock_4
Creating RawArray with float64 data, n_channels=39, n_times=31232
Range : 0 ... 31231 = 0.000 ... 124.924 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_0 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms3000 events and metadata
Creating RawArray with float64 data, n_channels=39, n_times=32768
Range : 0 ... 32767 = 0.000 ... 131.068 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_1 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms3000 events and metadata
Creating RawArray with float64 data, n_channels=39, n_times=31744
Range : 0 ... 31743 = 0.000 ... 126.972 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_2 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms3000 events and metadata
Creating RawArray with float64 data, n_channels=39, n_times=32512
Range : 0 ... 32511 = 0.000 ... 130.044 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_3 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms3000 events and metadata
Creating RawArray with float64 data, n_channels=39, n_times=28416
Range : 0 ... 28415 = 0.000 ... 113.660 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_4 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms3000 events and metadata
EEG data marked as already having the desired reference.
This sample mkh5 data file was created with coordinates for an idealized “average” head, based on 3D digitized locations. Head-shaped locations are called for when working with realistic head geometry in MNE but they don’t line up exactly with circular topographic maps.
_ = mne.viz.plot_sensors(mne_raw.info, sphere="auto")
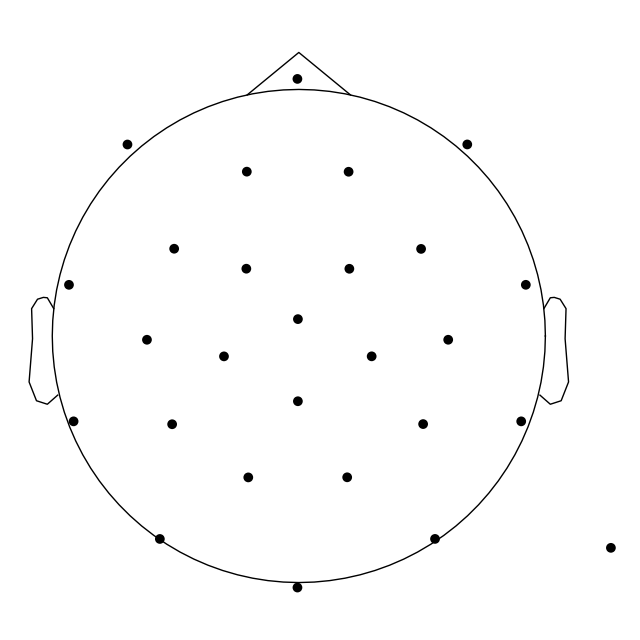
If original .yhdr did not include an apparatus map or a different set of locations is preferred, they can be specified when converting to MNE and used instead.
This map overrides the original head-shaped electrode locations with spherical 3D coordinates which are unrealistic as human head geometry but line up neatly with circles for painting pretty 2D pictures.
mne_raw_a = mkh5mne.from_mkh5(H5_F, apparatus_yaml="mne_32chan_xyz_spherical.yml")
_ = mne.viz.plot_sensors(mne_raw_a.info)
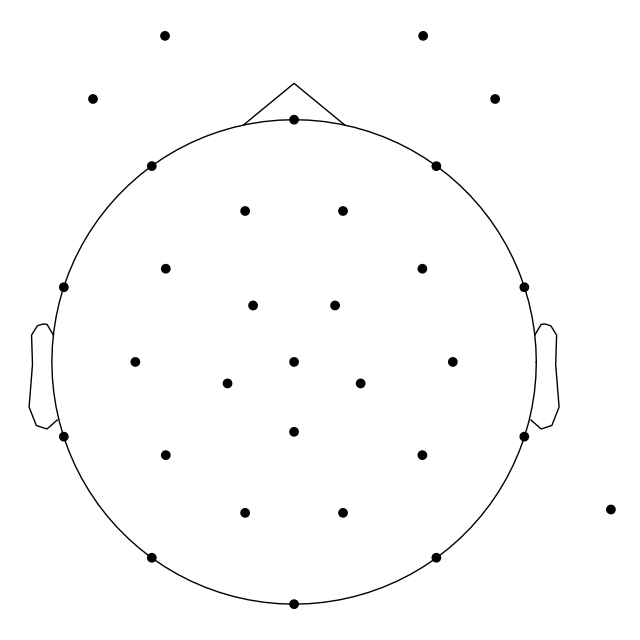
Out:
../mkh5_data/sub000p3.h5
looking up data block paths, larger files take longer ...
ok
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_0 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
checking info, montage sub000/dblock_1
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_1 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
checking info, montage sub000/dblock_2
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_2 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
checking info, montage sub000/dblock_3
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_3 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
checking info, montage sub000/dblock_4
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_4 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_0 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
Creating RawArray with float64 data, n_channels=39, n_times=31232
Range : 0 ... 31231 = 0.000 ... 124.924 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting position of 1 eog channel found in montage:
['HEOG']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_0 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms3000 events and metadata
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_1 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
Creating RawArray with float64 data, n_channels=39, n_times=32768
Range : 0 ... 32767 = 0.000 ... 131.068 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting position of 1 eog channel found in montage:
['HEOG']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_1 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms3000 events and metadata
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_2 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
Creating RawArray with float64 data, n_channels=39, n_times=31744
Range : 0 ... 31743 = 0.000 ... 126.972 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting position of 1 eog channel found in montage:
['HEOG']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_2 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms3000 events and metadata
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_3 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
Creating RawArray with float64 data, n_channels=39, n_times=32512
Range : 0 ... 32511 = 0.000 ... 130.044 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting position of 1 eog channel found in montage:
['HEOG']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_3 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms3000 events and metadata
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1190: UserWarning: Overriding /sub000/dblock_4 with sensor locations from mne_32chan_xyz_spherical.yml
warnings.warn(msg)
Creating RawArray with float64 data, n_channels=39, n_times=28416
Range : 0 ... 28415 = 0.000 ... 113.660 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting position of 1 eog channel found in montage:
['HEOG']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_4 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms3000 events and metadata
EEG data marked as already having the desired reference.
Selecting which datablocks to convert to mne.Raw
By default read_raw_mkh5()
converts the entire mkh5 file.
The mkh5 data groups and EEG datablocks are appended in the order
order returned by dblock_paths()
which sorts HDF5 the HDF5
paths to data group alphabetically and preserves data block order
within each group.
An mkh5 file might have multiple subjects or experiments, if you don’t want to make it all one mne.Raw object, you can
select which data blocks to convert with
datablock_paths=
.
mne_raw_b = mkh5mne.read_raw_mkh5(
H5_F, dblock_paths=["sub000/dblock_0", "sub000/dblock_1"]
)
# only dblock_0 and dblock_1 appear in the Raw data
mne_raw_b.annotations.description
Out:
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1688: UserWarning: mkh5mne.read_raw_mkh5() is deprecated and will be removed in a future release, use mkh5mn3.from_mkh5() instead
warnings.warn(
ok
checking info, montage sub000/dblock_1
Creating RawArray with float64 data, n_channels=39, n_times=31232
Range : 0 ... 31231 = 0.000 ... 124.924 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_0 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms3000 events and metadata
Creating RawArray with float64 data, n_channels=39, n_times=32768
Range : 0 ... 32767 = 0.000 ... 131.068 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_1 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms3000 events and metadata
EEG data marked as already having the desired reference.
array(['sub000/dblock_0', 'sub000/dblock_1', 'BAD boundary',
'EDGE boundary'], dtype='<U15')
Marking garv artifacts in mne.Raw
If you prepared the mkh5 file with the .log file log_flags set to
track garv artifacts (avg -x
) you can automatically mark the
garv artifacts in the mne.Raw with BAD_garv mne.Annotations by
passing in the garv_annotations
options.
# Mark *all* the log stim and response events events with lots of overlap
mne_raw_c = mkh5mne.from_mkh5(
H5_F,
garv_annotations={
"event_channel": "log_flags",
"tmin": -500,
"tmax": 1000,
"units": "ms",
},
)
Out:
../mkh5_data/sub000p3.h5
looking up data block paths, larger files take longer ...
ok
checking info, montage sub000/dblock_1
checking info, montage sub000/dblock_2
checking info, montage sub000/dblock_3
checking info, montage sub000/dblock_4
Creating RawArray with float64 data, n_channels=39, n_times=31232
Range : 0 ... 31231 = 0.000 ... 124.924 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_0 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'log_flags', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=32768
Range : 0 ... 32767 = 0.000 ... 131.068 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_1 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'log_flags', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=31744
Range : 0 ... 31743 = 0.000 ... 126.972 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_2 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'log_flags', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=32512
Range : 0 ... 32511 = 0.000 ... 130.044 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_3 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'log_flags', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=28416
Range : 0 ... 28415 = 0.000 ... 113.660 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_4 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'log_flags', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
EEG data marked as already having the desired reference.
Note
avg -x
flags all event codes in the log that fail a test whether or
not these are the timelocking events of interest.
annotation intervals for log events upstream or downstream of the
timelock of interest may overlap the epoch interval and
mne.Epochs(..., reject_by_annotation=True)
will exclude them for
being (partially) polluted. To avoid this, define your mkh5 codemap
and named epochs table so that epochs of interest do not overlap and
use that same-named MNE event channel to set the garv annoations
like so:
mne_raw_c = mkh5mne.from_mkh5(
H5_F,
garv_annotations={
"event_channel": "ms1500",
"tmin": -500,
"tmax": 1000,
"units": "ms",
},
)
mne_raw_c.plot(scalings={"eeg": 5e-5, "eog": 1e-4}, start=10.0, n_channels=39)
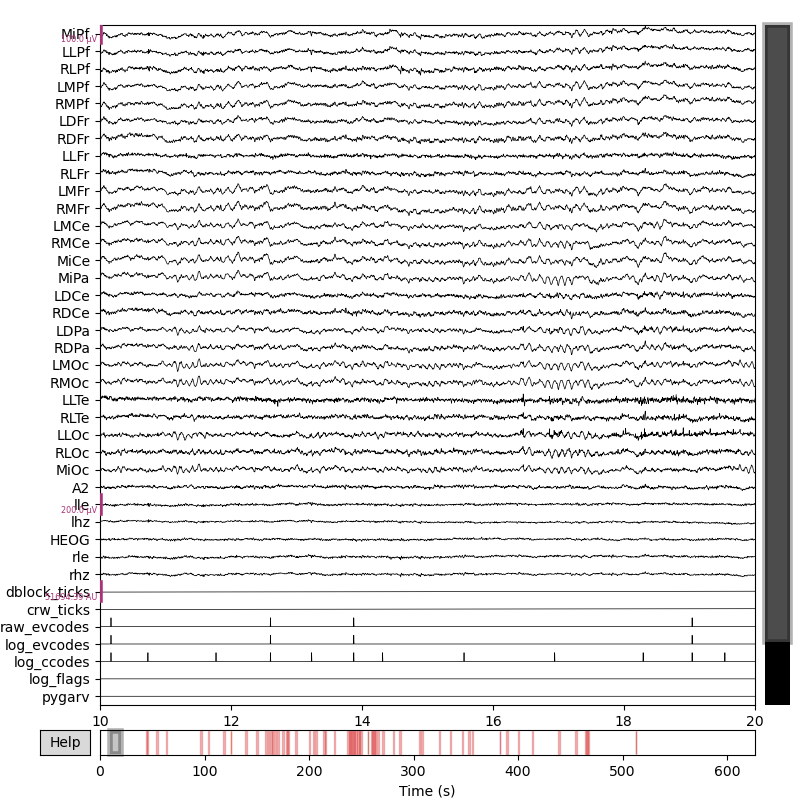
Out:
../mkh5_data/sub000p3.h5
looking up data block paths, larger files take longer ...
ok
checking info, montage sub000/dblock_1
checking info, montage sub000/dblock_2
checking info, montage sub000/dblock_3
checking info, montage sub000/dblock_4
Creating RawArray with float64 data, n_channels=39, n_times=31232
Range : 0 ... 31231 = 0.000 ... 124.924 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_0 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_0 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'ms1500', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=32768
Range : 0 ... 32767 = 0.000 ... 131.068 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_1 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_1 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'ms1500', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=31744
Range : 0 ... 31743 = 0.000 ... 126.972 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_2 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_2 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'ms1500', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=32512
Range : 0 ... 32511 = 0.000 ... 130.044 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_3 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_3 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'ms1500', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
Creating RawArray with float64 data, n_channels=39, n_times=28416
Range : 0 ... 28415 = 0.000 ... 113.660 secs
Ready.
/usr/share/miniconda/envs/env_3.8/lib/python3.8/site-packages/mkpy/io/mkh5mne.py:1478: RuntimeWarning: Not setting positions of 5 eog channels found in montage:
['lle', 'lhz', 'HEOG', 'rle', 'rhz']
Consider setting the channel types to be of EEG/sEEG/ECoG/DBS/fNIRS using inst.set_channel_types before calling inst.set_montage, or omit these channels when creating your montage.
raw_dblock.set_montage(montage)
sub000/dblock_4 setting mkh5 epochs table ms100 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms10000 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms1500 events and metadata
sub000/dblock_4 setting mkh5 epochs table ms3000 events and metadata
annotating garv artifacts {'event_channel': 'ms1500', 'tmin': -500, 'tmax': 1000, 'units': 'ms'}
EEG data marked as already having the desired reference.
Opening raw-browser...
<MNEBrowseFigure size 800x800 with 4 Axes>
Total running time of the script: ( 1 minutes 32.366 seconds)